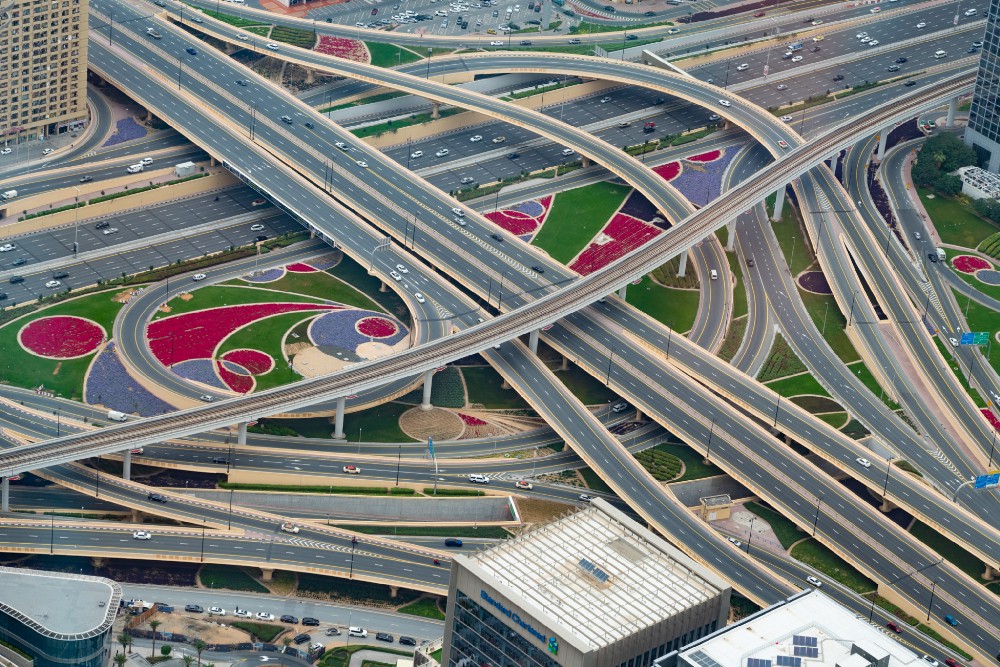
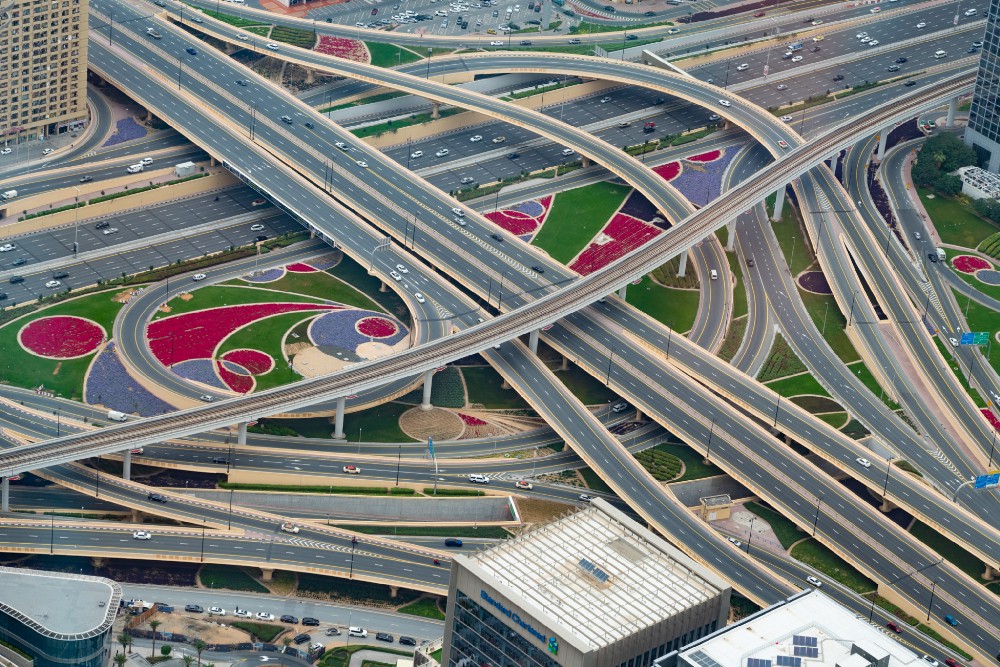
This tutorial will go through the steps to set up routing with express.js and some best practices.
This post follows the setup of the Express.js backend system described here: How to set up a Node.js backend using Express.js and TypeScript
What is routing?
A route is a system that associates an HTTP request and a function that will execute a process. There can be some parameters to handle, and the function can provide an answer to send back to the process requesting the route.
How to create a simple route?
In the index.tx file located in the src folder, we will add our first route :
app.get('/', (req, res) => {
res.send("What's up doc ?!");
});
This code means :
the URL that will request the root route with a GET method will trigger a function that will send the message “What’s up doc ?!”
Insert this code in the index.ts file :
import express from 'express';
import dotenv from 'dotenv';
const app = express();
dotenv.config();
// routes
app.get('/', (req, res) => {
res.send("What's up doc ?!");
});
// start the server
app.listen(process.env.BACK_PORT, () => {
console.log(
`server running : http://${process.env.BACK_HOST}:${process.env.BACK_PORT}`
);
});
and run the server with the terminal command
npm run start
Then with a web browser, enter the URL of the server. In my case : http://localhost:8080
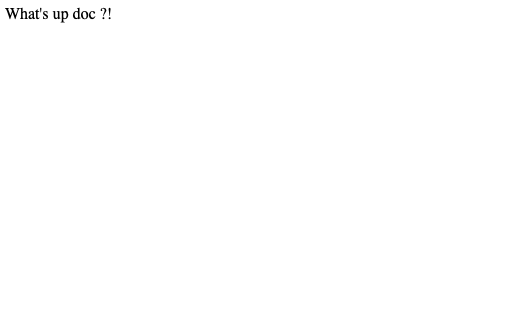
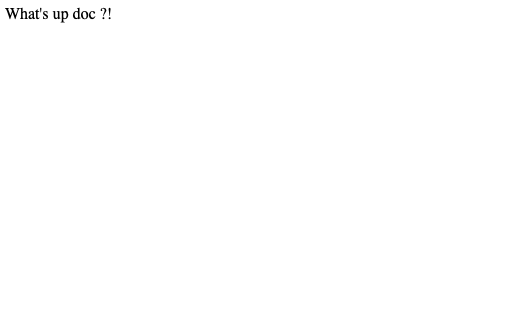
So now the root route “/” returns the message “What’s up doc ?!”
Manage routing in separate files
We could handle all the routes in the main index.ts file and list all of them with the functions and processes, but splitting them into several files will help us have a more maintainable code.
So in the src folder, let’s create a routes folder and a defaultRoute.ts file inside it.
We will now declare the root route in defaultRoute.ts like this:
import { Router } from 'express';
export const defaultRoute = Router();
defaultRoute.get('/', (req, res) => {
res.send("What's up doc ?!");
});
This code looks very similar to the one we wrote in the index.ts.
Now, inside the routes filter, create an index.ts file that will gather all routes from the different files:
import express from 'express';
import { defaultRoute } from './defaultRoute';
export const routes = express.Router();
routes.use(defaultRoute);
And finally, let’s update the src/index.ts to use these routes :
import express from 'express';
import dotenv from 'dotenv';
import { routes } from './routes';
const app = express();
dotenv.config();
// routes
app.use('/', routes);
// start the server
app.listen(process.env.BACK_PORT, () => {
console.log(
`server running : http://${process.env.BACK_HOST}:${process.env.BACK_PORT}`
);
});
Stop and run the server again to see that you have the same result as before.
From here we can start building our routes.
Route with process
Let’s do something more funny: we will create a route that takes 2 numbers as parameters and return the sum.
As we will expect some data, we will use the POST method and listen for the route /calc.
First, in src/routes, we will create a calcRoute.ts with a new route listening for a post method on the /calc URL:
import express, { Request, Response } from 'express';
export const calcRoute = express.Router();
calcRoute.post('/calc', (req: Request, res: Response) => {
});
And now we add the process:
- check if both the parameters exist in the request body
- return the sum in a json format
- return an error in a json format if a parameter is missing or is not a number
import express, { Request, Response } from 'express';
export const calcRoute = express.Router();
calcRoute.post('/calc', (req: Request, res: Response): void => {
const { a, b } = req.body;
if (a && b && typeof a === 'number' && typeof b === 'number') {
res.json({
success: true,
message: a + b,
});
} else {
res.json({
success: false,
message: 'Missing parameters',
});
}
});
To get this route properly working we need to:
- add it to the routes/index.ts
- install body-parser to get the parameters from the request
Adding the route
In the src/routes/index.ts we will add the new route:
import express from 'express';
import { calcRoute } from './calcRoute';
import { defaultRoute } from './defaultRoute';
export const routes = express.Router();
routes.use(defaultRoute);
routes.use(calcRoute);
Installing and setup body-parser
In the terminal, let’s install the library
npm install body-parser --save
In the src/index.ts we will set it up:
import express from 'express';
import { Application } from 'express';
import dotenv from 'dotenv';
import { routes } from './routes';
import bodyParser from 'body-parser';
const app: Application = express();
// body-parser
app.use(bodyParser.json({ limit: '50mb', type: 'application/json' }));
app.use(bodyParser.urlencoded({ limit: '50mb', extended: true }));
dotenv.config();
// routes
app.use('/', routes);
// start the server
app.listen(process.env.BACK_PORT, () => {
console.log(
`server running : http://${process.env.BACK_HOST}:${process.env.BACK_PORT}`
);
});
Test the route
To test this route we will need a software like postman to send the request with the parameters in the body after restarting the server:
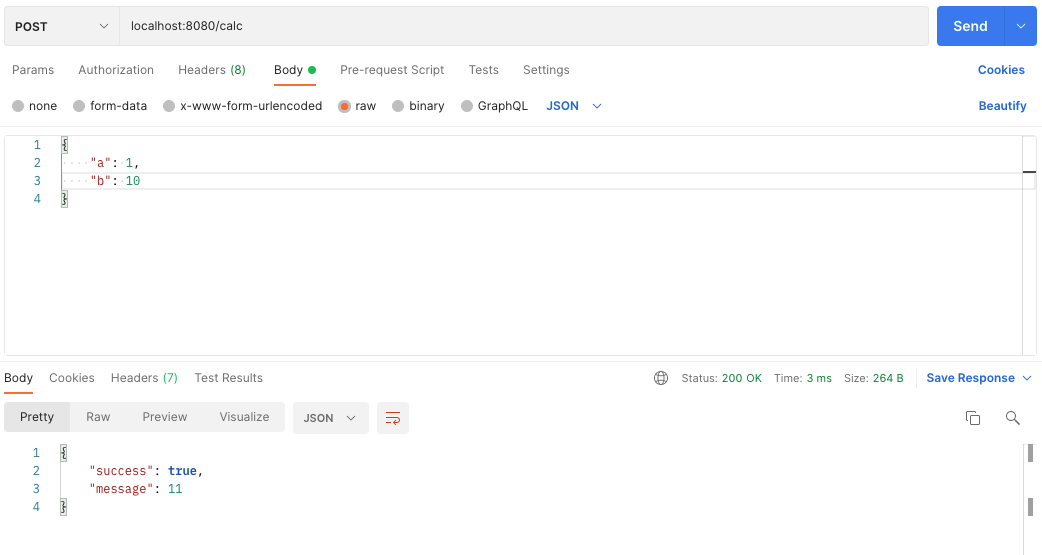
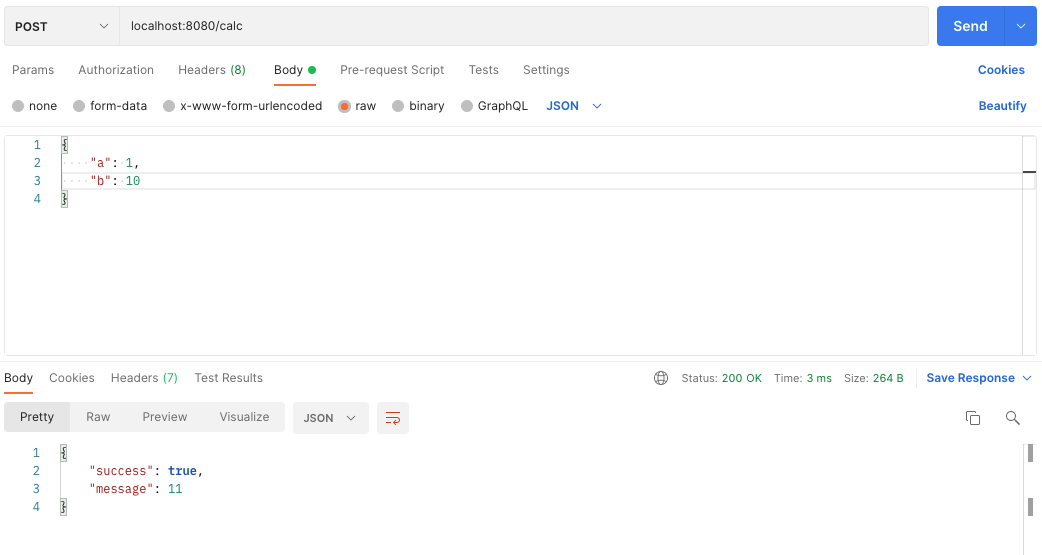
Conclusion
We looked at how to route and handle them in distinct files in this brief example. There’s still much more to say about routes, particularly when it comes to more complicated use cases and the use of middlewares to manage processes.
After reading this document, I hope you will have a clear understanding of the basics of express.js’s routing system.
What can you do to help me?
Please don’t hesitate to:
- Like the article
- Follow me
- Leave a comment and express your opinion.
Happy coding!