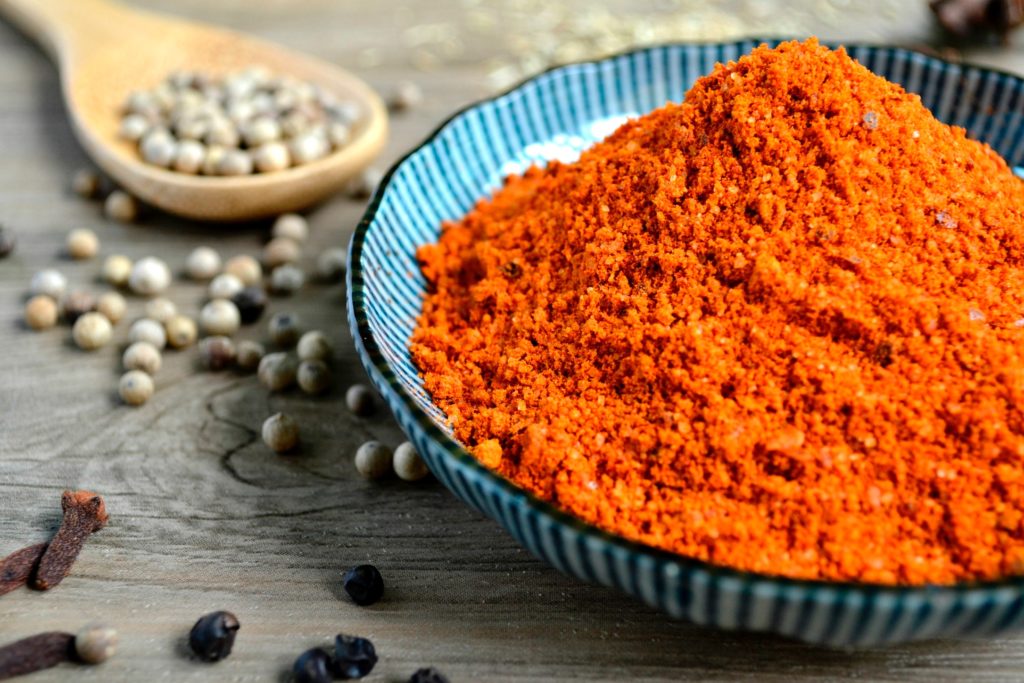
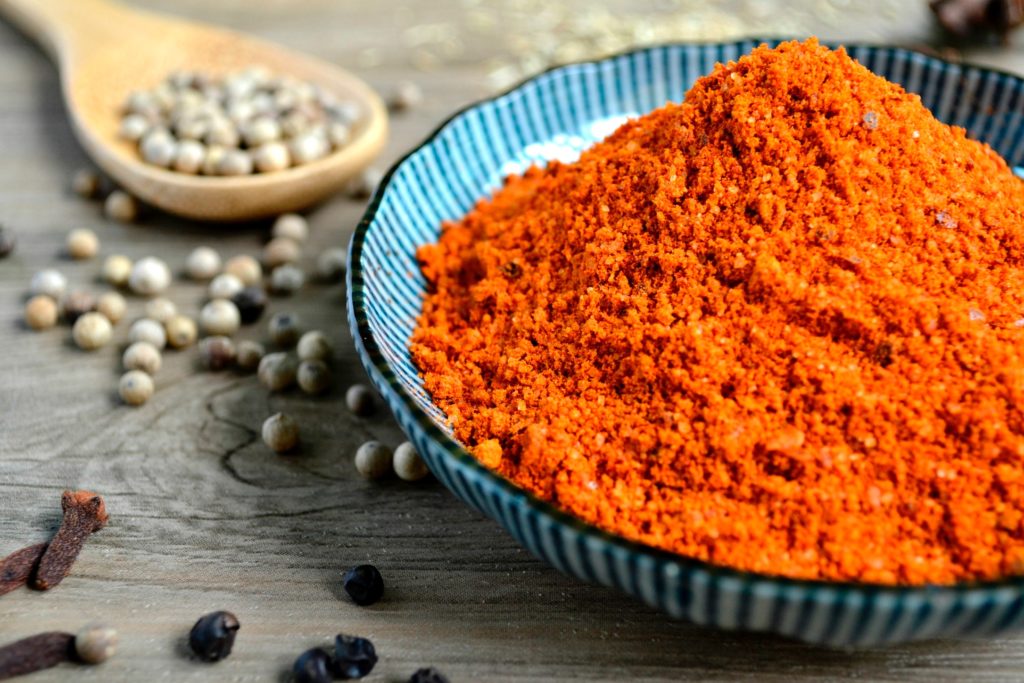
Currying is probably one of the notions I had the most trouble understanding when developing in javascript.
What is currying?
First, it has nothing to do with a spice or exotic cooking!
Wikipedia defines it as follows:
In computer science, more precisely in functional programming, currying transforms a function with several arguments into a function with one argument that returns a function on the rest of the arguments. The term comes from the name of the American mathematician Haskell Curry.
The principle is to decompose a function that takes n parameters into n functions that take 1 parameter.
This also allows working with pure functions, a fundamental principle of functional programming.
Example
Let’s take, for example, a function that adds two integers, 1 and 2, and that will return 3 :
const addition = (x, y) => x + y;
const result = addition(1, 2);
console.log(result); // 3
If we now apply the currying method to this function, we will declare a function that will return a function that will take the second argument, and that will return the result of the addition:
const addition = (x) => (y) => x + y;
const result = addition(1)(2);
console.log(result); // 3
Apart from the syntax, there is no significant advantage, and that’s where I got stuck understanding the principle well.
With the currying, it will be possible to make partial use of the function and thus compose others. With our basic function, we will create a new function which will add 3 to an integer passed in parameter:
const addition = (x) => (y) => x + y;
const addThree = addition(3)
The function add3() is a partial use of the function addition and to finish the example:
const addition = (x) => (y) => x + y;
const addThree = addition(3);
const result = addThree(5);
const result2 = addThree(2);
console.log(result); // 8
console.log(result2); // 5
In our example, writing: addThree(5)
is, in fact, the same as writing: addition(3)(5)
Hence the power of the partial use of a function to create new ones quickly and easily.
Conclusion
The currying is, in my opinion, a powerful tool for composing functions in javascript. I even use it outside of functional programming to simplify and factorize the code of some functions.
What can you do to help me?
Please don’t hesitate to:
- Like the article
- Follow me
- Leave a comment and express your opinion.
Happy coding!