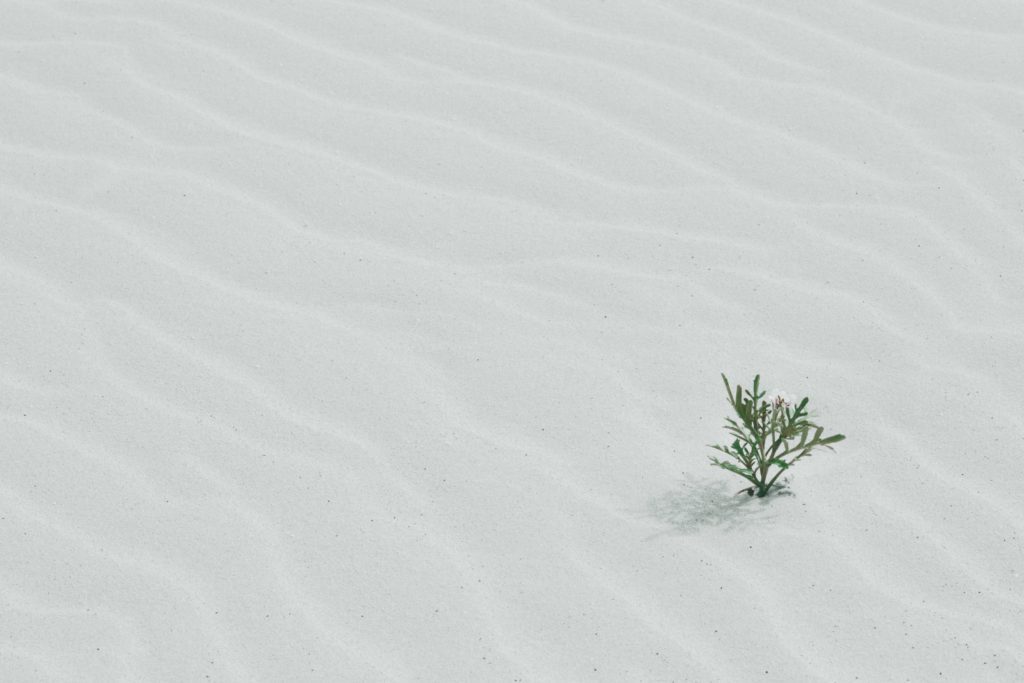
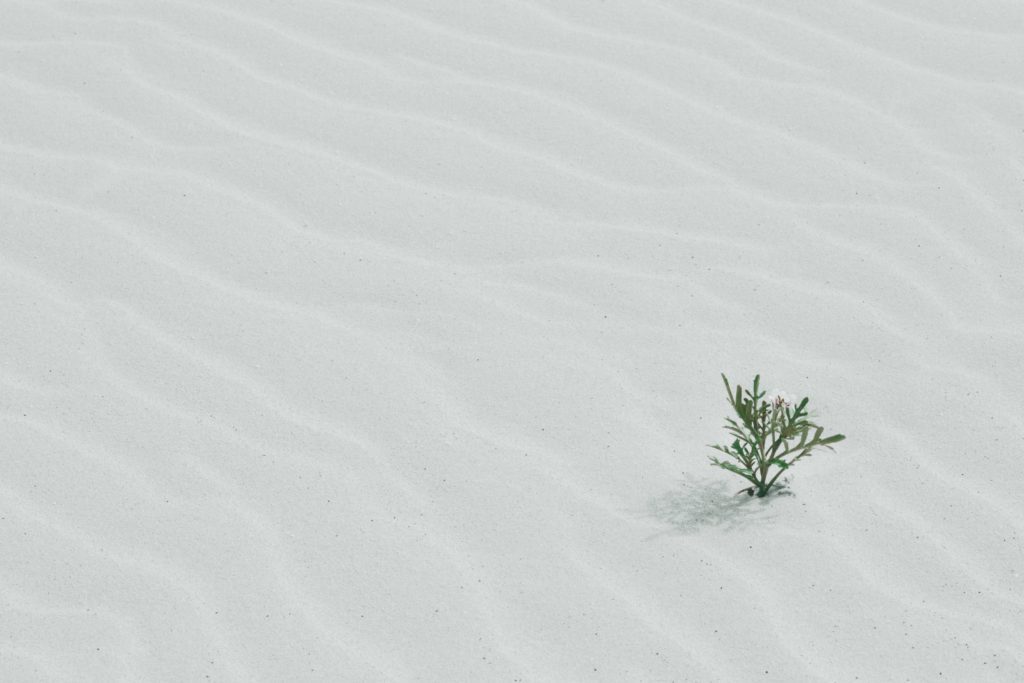
What are pure functions? In short, pure functions don’t modify the global state and don’t depend on any external input. They always return the same result when given the same input. This makes them predictable and reliable. This blog post will discuss the benefits of using pure functions in JavaScript and show you how to write your own!
Benefits
One of the biggest benefits of using pure functions is that they are predictable and reliable. When you call a pure function, you can be sure that it will always return the same result, given the same input. This makes them perfect for use in libraries or frameworks, where you need to be able to count on their behavior. Pure functions are also easy to debug, since any errors will be caused by the function itself, and not by some external input.
Another benefit of pure functions is that they are lightweight. They don’t require any special libraries or frameworks, and they can be executed in any environment. This makes them a great choice for small scripts or prototypes.
Pure functions also have some performance benefits. Because they don’t modify the global state, they are faster than regular functions. They also use less memory, which is important for applications with large data sets.
Finally, pure functions are simple and easy to understand. You don’t need to know anything about the internals of a function to use it correctly. This makes them ideal for beginners who are just learning JavaScript.
So, how can you write pure functions?
It’s very easy! Just follow these simple steps:
- First, create a function that takes one input and returns a result.
- Next, make sure the function doesn’t modify the global state or depend on any external input.
- Finally, test the function to make sure it returns the same result every time.
Here’s an example of a pure function in action:
// return the sum of 2 numbers
const getSum = (a, b) => {
return a + b;
};
console.log(getSum(1, 2)); // returns 3
And on the other hand, here is an example of an impure function:
// return the sum of 2 numbers and add a parameter value
const addParameter = 3;
const getSumPlusParameter = (a, b) => {
return a + b + addParameter;
};
console.log(getSumPlusParameter(1, 2)); // returns 6 and depends of an external parameter
When to use pure functions?
- When you need a function to always return the same result, given the same input. Pure functions are perfect for this purpose.
- When you’re writing a library or framework that will be used by other developers. Pure functions are the best way to ensure predictable and reliable behavior.
- When you’re learning JavaScript. Pure functions are simple and easy to understand, making them ideal for beginners.
And … when to use impure functions?
- When you need to call a function with side effects (such as modifying global state or accessing external resources)
- When the function is too complex to be written as a pure function
- When performance is more important than reliability or readability.
Impure functions can be a bit more tricky to debug than pure functions, but they often have better performance. If you need to call a function with side effects, or if the function is too complex to be written as a pure function, then it’s best to use an impure function. Just make sure you test it thoroughly and be aware of any potential problems!
Conclusion
- When in doubt, use a pure function. Pure functions are easier to read and maintain, and they always return the same result.
- Use impure functions when you need to call a function with side effects, or when the function is too complex to be written as a pure function. Be aware of any potential problems that may arise
As you can see, using pure functions in JavaScript is a great way to improve reliability and performance while keeping things simple and easy to understand. So, next time you’re writing a script or prototype, be sure to use pure functions! They’ll make your code easier to read and maintain.
What can you do to help me?
Please don’t hesitate to:
- Like the article
- Follow me
- Leave a comment and express your opinion.
Happy coding! 🙂