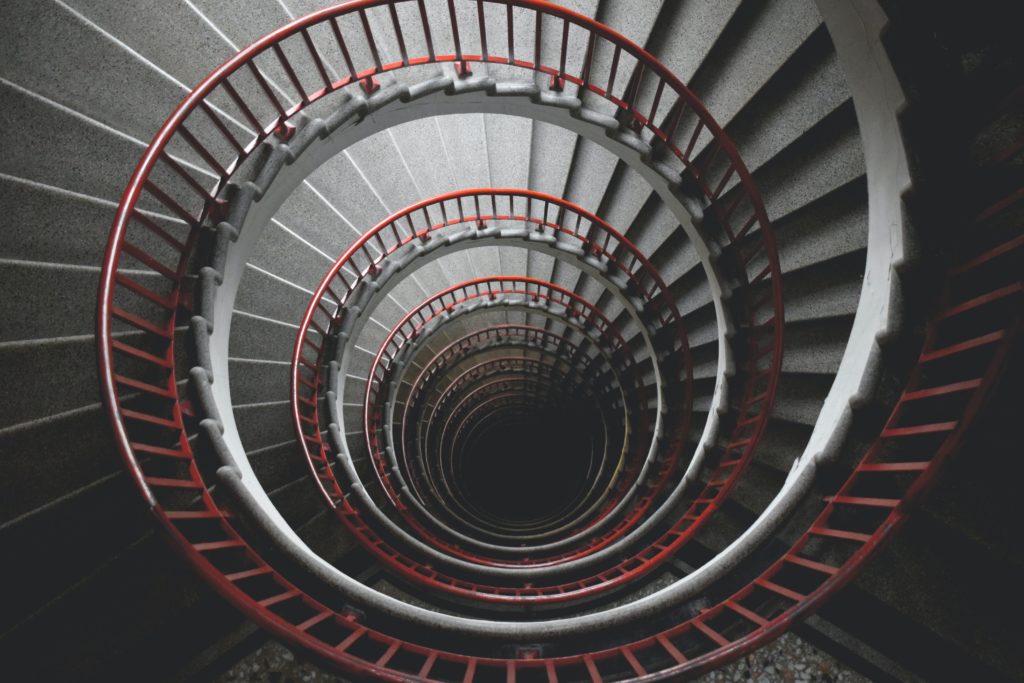
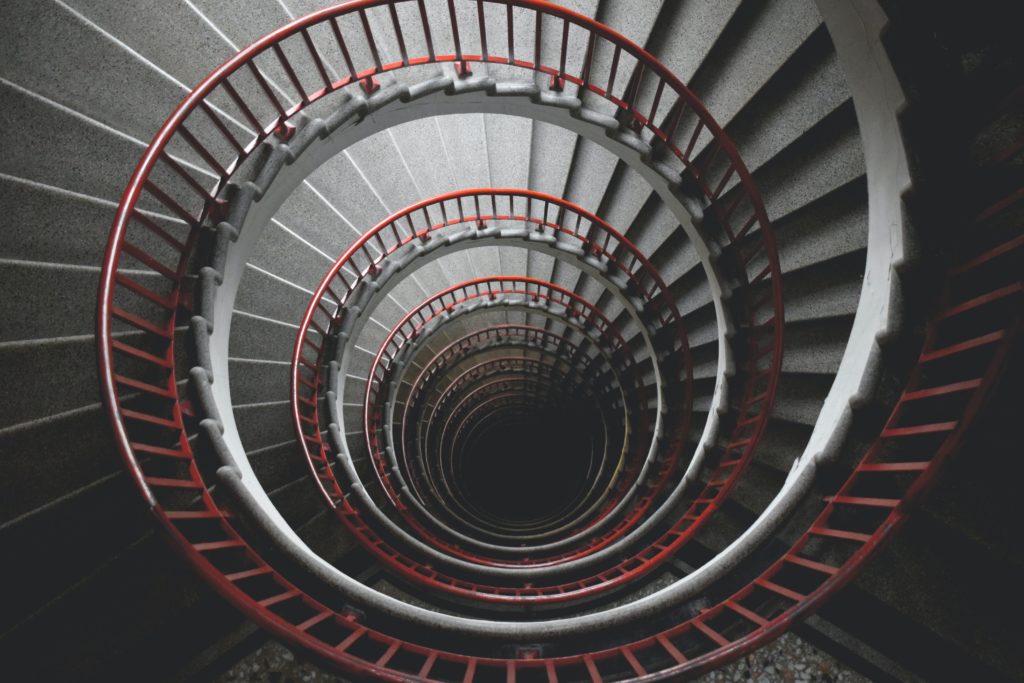
Javascript is a programming language originally designed for web development. It has been standardized through the ECMAScript language specification and can be used to create both mobile and desktop applications. Javascript is one of the most popular programming languages in use today, powering everything from simple scripts on your website to complex programs that run inside the software.
One of the most common tasks you’ll need to perform when working with Javascript is looping through an array. This can be done in several different ways, each with its trade-offs and benefits. In this article, we’ll explore 5 different ways to iterate over an array in Javascript:
for loop
A for loop is the most traditional way of looping through an array. It looks like this:
for (var i = 0; i < array.length; i++) {
// do something with array element at index i
};
This code will loop through every element in the array and do something with it. The variable i is used as a counter, starting at 0 and going up to (but not including) the array’s length. You can use this variable inside the loop to access each element of the array in turn.
One advantage of a for loop is that it’s easy to set up and use. You can also add additional conditions inside the for loop’s parentheses to control how many times the loop runs. For example, you could change the loop to only run 10 times by doing this:
for (var i = 0; i < 10; i++) {
// do something with array element at index i
};
Another advantage of a for loop is that it’s easy to nest inside another for loop to iterate over multiple arrays at the same time. For example, if you had two arrays, arr1
and arr2
, and you wanted to loop through both of them at the same time, you could do this:
for (var i = 0; i < arr1.length; i++) {
for (var j = 0; j < arr2.length; j++) {
// do something with array element at index i from arr1
// and array element at index j from arr2
}
}
However, there are some disadvantages to using a for loop. One is that it can be easy to make mistakes when writing the code inside the loop. For example, if you accidentally forget to increase the value of the counter variable (i++
), the loop will run forever and crash your program. Another disadvantage is that for loops can be harder to read and understand than other ways of looping through arrays.
forEach loop
A forEach loop is a newer way of iterating over arrays that was introduced in Javascript 1.6. It looks like this:
array.forEach(element => {
// do something with element
});
This code will loop through every element in the array and do something with it. The forEach loop will call the function you provide once for each element in the array, passing in the element as an argument. You can then use this argument inside the function to perform whatever operation you want on the element.
One advantage of a forEach loop is that it’s more concise and easier to read than a for loop. It can also be easier to use since you don’t have to remember to increment the counter variable (i++
). Another advantage is that forEach loops are closer in syntax to other languages like C# and Java, which can make them easier to understand for people who come from those backgrounds.
However, there are some disadvantages to using a forEach loop. One is that you can’t break out of a forEach loop early like you can with a for loop. This means that if you have a large array and you only want to loop through the first 10 elements, you can’t do this with a forEach loop. Another disadvantage is that forEach loops can be slower than for loops, since they have to call the function you provide for each element in the array.
map function
The map function is a way of iterating over an array and returning a new array with the results of calling a function on each element. It looks like this:
var newArray = array.map(element => {
return // something;
});
This code will create a new array by calling the function you provide for each element in the array. The function you provide should return the value you want to store in the new array at the corresponding index. For example, if you wanted to square each element in an array, you could do this:
var arr = [-2, -1, 0, 1, 2];
var squaredArr = arr.map(element => {
return element * element;
});
console.log(squaredArr); // => [4, 1, 0, 1, 4]
This code will create a new array called squaredArr
with the results of squaring each element in the arr
array. Note that we’re using the map function to avoid changing the original arr
array (which is good practice).
Advantages and disadvantages are the same as the forEach function.
filter function
The filter function is a way of iterating over an array and returning a new array with only the elements that meet certain criteria. It looks like this:
var newArray = array.filter(element => {
return // some condition;
});
This code will create a new array by calling the function you provide for each element in the array. The function you provide should return true if the element should be included in the new array, and false if it should not. For example, if you wanted to get only the positive numbers from an array, you could do this:
var arr = [-2, -1, 0, 1, 2];
var positiveArr = arr.filter(element => {
return element > 0;
});
console.log(positiveArr); // => 1, 2
This code will create a new array called positiveArr
that includes only the elements from arr
that are greater than 0. Note that we’re using the filter function to avoid changing the original arr
array (which is good practice).
Advantages and disadvantages are the same as the forEach function.
reduce function
The reduce function is a way of iterating over an array and reducing it to a single value. It looks like this:
var newValue = array.reduce((accumulator, element) => {
return // some operation on accumulator and element;
}, initialValue);
This code will create a new value by calling the function you provide for each element in the array. The function you provide should take two arguments: an accumulator and an element. The accumulator is the running total of all the values that have been returned so far, and the element is the current element being iterated over. For example, if you wanted to sum all the values in an array, you could do this:
var arr = [-2, -1, 0, 1, 2];
var sum = arr.reduce((accumulator, element) => {
return accumulator + element;
}, 0); // initialValue is 0
console.log(sum); // => 0
This code will create a new value called sum which is the sum of all the values in arr. Note that we’re using the reduce function to avoid changing the original arr
array (which is good practice).
One advantage of using the reduce function is that you can use it to quickly get a single value from an array without having to loop through the entire array. This can be much faster than iterating over the array with a for loop. Another advantage is that you can provide an initialValue as the second argument, which can be helpful in certain situations.
However, there are some disadvantages to using the reduce function. One is that it can be more difficult to understand than a for loop. This is because the syntax is more complex and it can be difficult to keep track of the accumulator variable. Another disadvantage is that you can’t break out of a reduce function early like you can with a for loop. This means that if you have a large array and you only want to iterate through the first 10 elements, you can’t do this with the reduce function.
Conclusion
There are many ways to iterate over an array in Javascript. The most common way is with a for loop, but there are also forEach, map, filter, and reduce functions that can be used. Each of these has its advantages and disadvantages, so it’s important to choose the right one for the task at hand. In general, for loops are the most versatile and easy to understand, but the other functions can be faster and more concise in certain situations.
What can you do to help me?
Please don’t hesitate to:
- Like the article
- Follow me
- Leave a comment and express your opinion.
Happy coding!