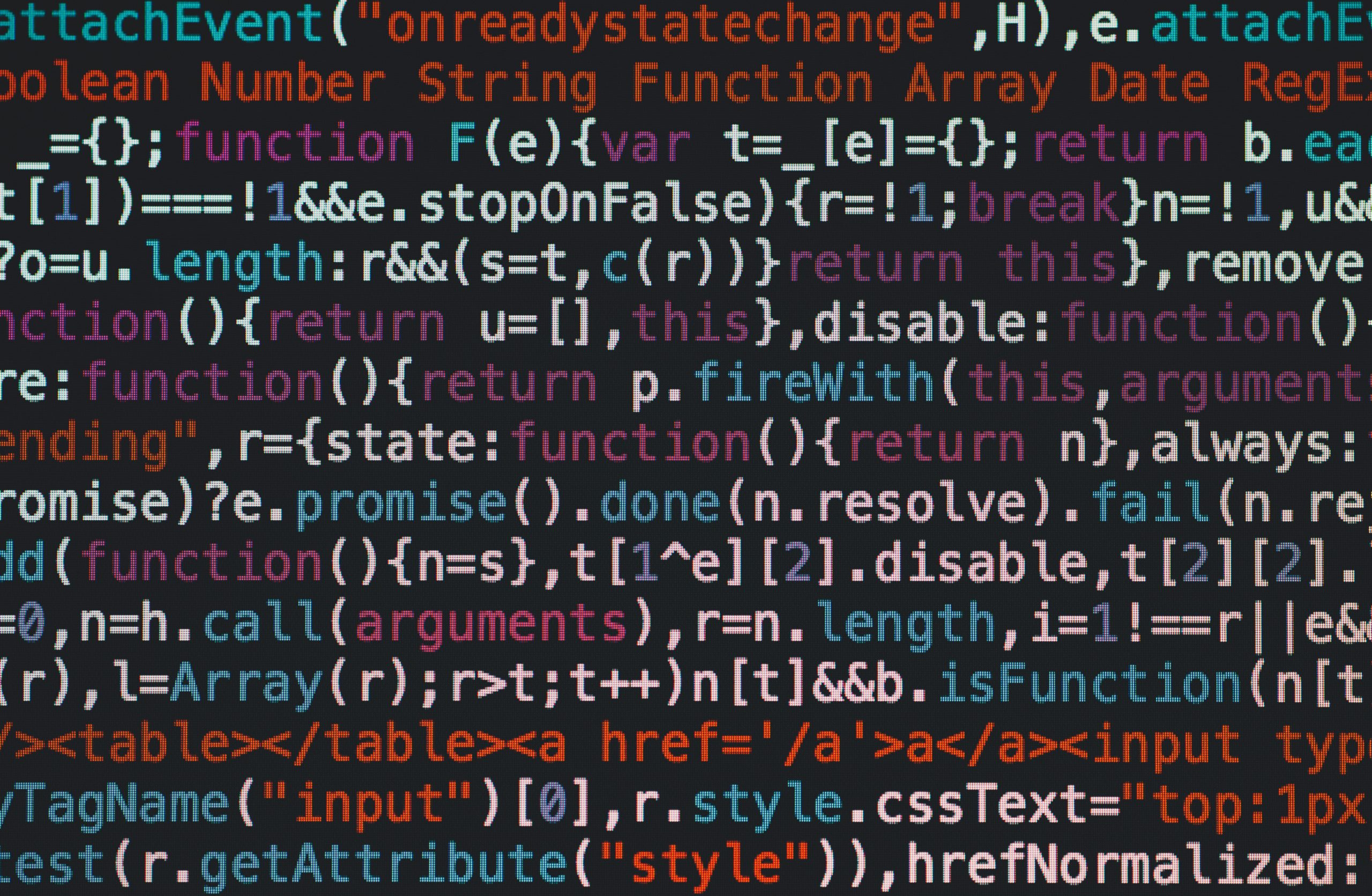
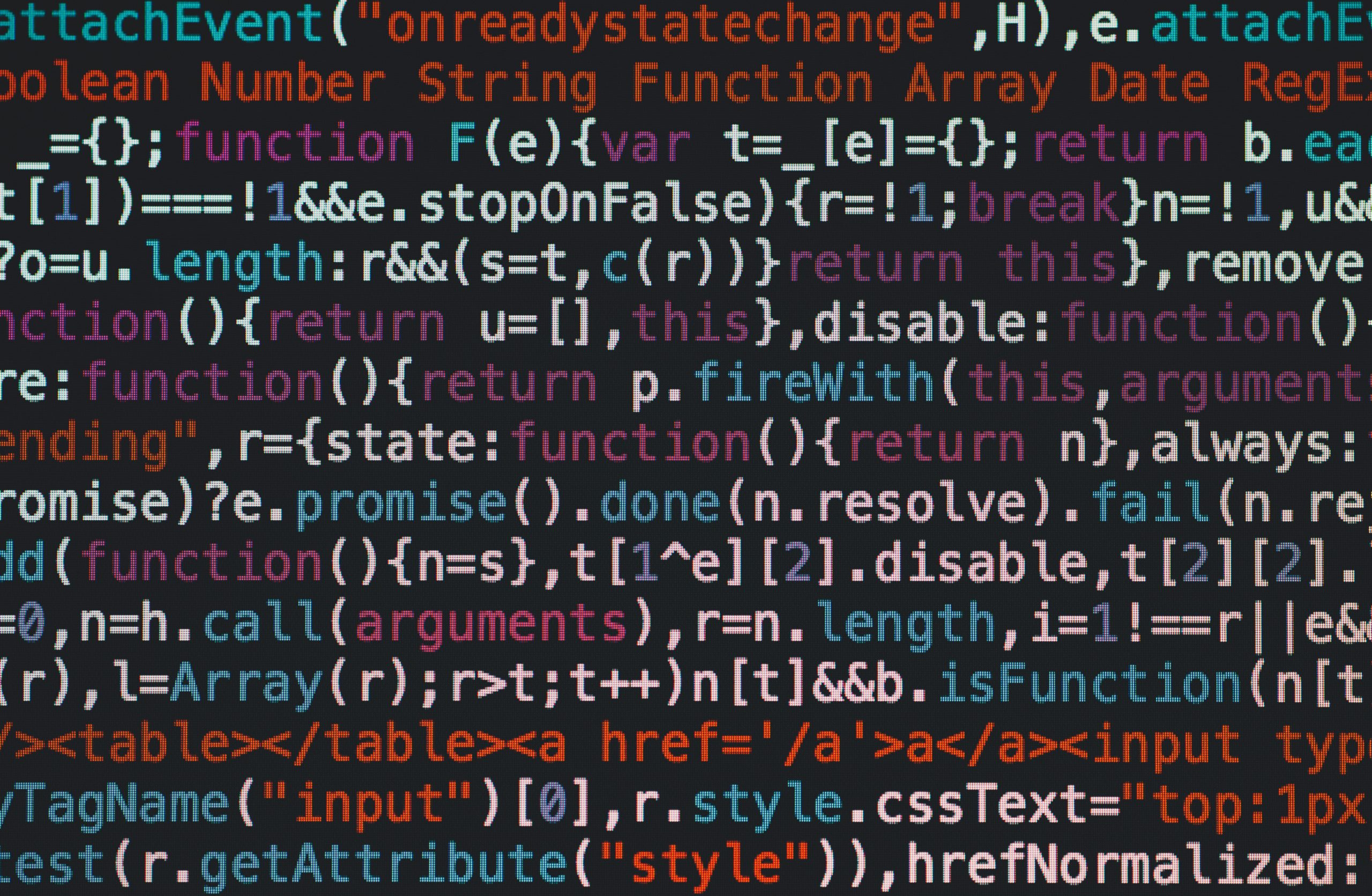
Regular expressions, also known as “regex” or “regexp”, are a powerful tool used for pattern matching in strings. They are a sequence of characters that define a search pattern, and are used in various programming languages, including JavaScript, Python, Perl, …, to perform tasks such as search and replace, validation, and data extraction.
A regular expression consists of a combination of characters and special symbols, such as .
, *
, +
, ?
, []
, {}
, |
, and ^
. These symbols have special meanings and are used to define the search pattern. For example, the .
symbol is used to match any character, the *
symbol is used to match zero or more occurrences of the preceding character, and the []
symbol is used to match a range of characters.
The purpose of regular expressions is to provide a concise and flexible means to match strings, or parts of strings, against a pattern. They are particularly useful when working with large amounts of text data, such as log files, CSV files, or HTML documents.
Main operators
- Literal characters: These are the basic building blocks of regular expressions and match themselves exactly. For example, the regular expression “a” would match only the letter “a”. Literal characters are case sensitive.
- Anchors: These operators specify the position of the regular expression in the text being searched. For example, the “^” operator matches the start of a line and the “$” operator matches the end of a line.
- Quantifiers: These operators specify how many times a character or group of characters should be matched. For example, the “” operator matches zero or more occurrences of the preceding character or group, and the “+” operator matches one or more occurrences.
- Character classes: These operators allow you to match any one character from a set of characters. For example, the “abc” operator would match any one of the letters “a”, “b”, or “c”.
- Alternation: This operator allows you to match one of several possible patterns. For example, the “|” operator separates two or more alternatives, and the regular expression “a|b” would match either “a” or “b”.
- Grouping: This operator allows you to group parts of the regular expression together. For example, the “( )” operator groups characters together.
- Special sequences: These are special sequences of characters that have a special meaning in regular expressions. For example, the “d” operator matches any digit, and the “w” operator matches any word character.
- Back-references: These operators allow you to reuse the text matched by a previous capturing group. For example, the “1” operator would match the same text as the first capturing group.
Writing regular expressions
Writing regular expressions can seem daunting at first, but with a bit of practice, it can become a very useful skill. Here are some tips and guidelines on how to write regular expressions:
- Start with a simple pattern: When writing a regular expression for the first time, it’s best to start with a simple pattern that you want to match. This could be a single word, a sequence of numbers, or a specific character. Once you have a simple pattern that works, you can start to build on it and make it more complex.
- Use special characters sparingly: Regular expressions have a variety of special characters that can be used to match different types of patterns. However, it’s important to use them sparingly and only when necessary. Overuse of special characters can make your regular expression difficult to read and understand.
- Test your regular expression: Before using your regular expression in your code, it’s a good idea to test it to make sure it works as expected. There are many online tools that you can use to test regular expressions, such as regex101.com, which allows you to test a regular expression against a string and see the result.
- Use the right method: Different languages have different methods of working with regular expressions. In javascript you have
test()
,exec()
,match()
,search()
,replace()
, andsplit()
which are used for different purposes, make sure to choose the right method for the task you want to perform. - Use Anchors: Anchors are special characters that match the position of a string rather than the characters themselves. For example,
^
matches the start of a string, and$
matches the end of a string. These are useful when you want to match a pattern at the beginning or end of a string. - Use grouping and alternation: Regular expressions allow you to group characters together and use alternation to match multiple patterns. For example, you can use the
|
character to match multiple patterns, such as(cat|dog)
to match either “cat” or “dog”. - Use quantifiers: You can use quantifiers to match specific numbers of characters, such as
*
to match zero or more occurrences,+
to match one or more occurrences, and?
to match zero or one occurrences.
By following these tips, you should be able to write regular expressions that are efficient and easy to understand. Remember that regular expressions can be complex and it takes time and practice to master them. It’s always a good idea to test your regular expressions thoroughly and make sure they work as expected.
Examples and use cases of regular expressions in JavaScript:
Validate an email address:
let email = "email@example.com";
let emailRegex = /^[a-zA-Z0-9._-]+@[a-zA-Z0-9.-]+\.[a-zA-Z]{2,}$/;
let isValid = emailRegex.test(email);
console.log(isValid); // true
This code will check if the email address is valid using a regular expression that checks for the presence of an alphanumeric username, an @ symbol, a domain name, and a valid top-level domain.
Search and replace all occurrences of a word in a string:
let string = 'This is an example string.';
let replaced = string.replace(/an example/g, 'a test');
console.log(replaced); // "This is a test string."
This code will replace all occurrences of the word “an example” with « a test” using the replace()
method.
Extracting information from a string:
let string = "The phone number is 555-555-5555.";
let match = string.match(/\d{3}-\d{3}-\d{4}/);
console.log(match[0]); // "555-555-5555"
This code will extract the phone number from the string using the match()
method and a regular expression that looks for a pattern of three digits, a dash, three digits, a dash, and four digits.
Use Case 1 : Data validation and sanitization
Regular expressions are useful for validating and sanitizing user input, such as email addresses, phone numbers, and credit card numbers. By using regular expressions to define a pattern that must be matched, you can ensure that the data entered by the user is in the correct format, and remove any unwanted characters or illegal input.
Use Case 2 : Text processing and data extraction
Regular expressions are also useful for text processing and data extraction. You can use regular expressions to extract specific pieces of information from a large block of text, such as email addresses, phone numbers, URLs, or dates. This can be useful when working with log files, CSV files, or HTML documents and looking for specific data or patterns.
Conclusion
Regular expressions are a powerful tool that can be used to perform a wide variety of tasks related to pattern matching in strings. They are particularly useful when working with large amounts of text data, such as log files, CSV files, or HTML documents. Regular expressions can be used for data validation and sanitization, text processing and data extraction, and many more. With the right combination of characters and special symbols, regular expressions can help you to define a search pattern that can be used to match, extract or replace specific parts of a string. It’s a skill that’s well worth learning if you’re working with text data and want to automate your text-processing tasks.
What can you do to help me?
Please don’t hesitate to:
- Like the article
- Follow me
- Leave a comment and express your opinion.
Happy coding!