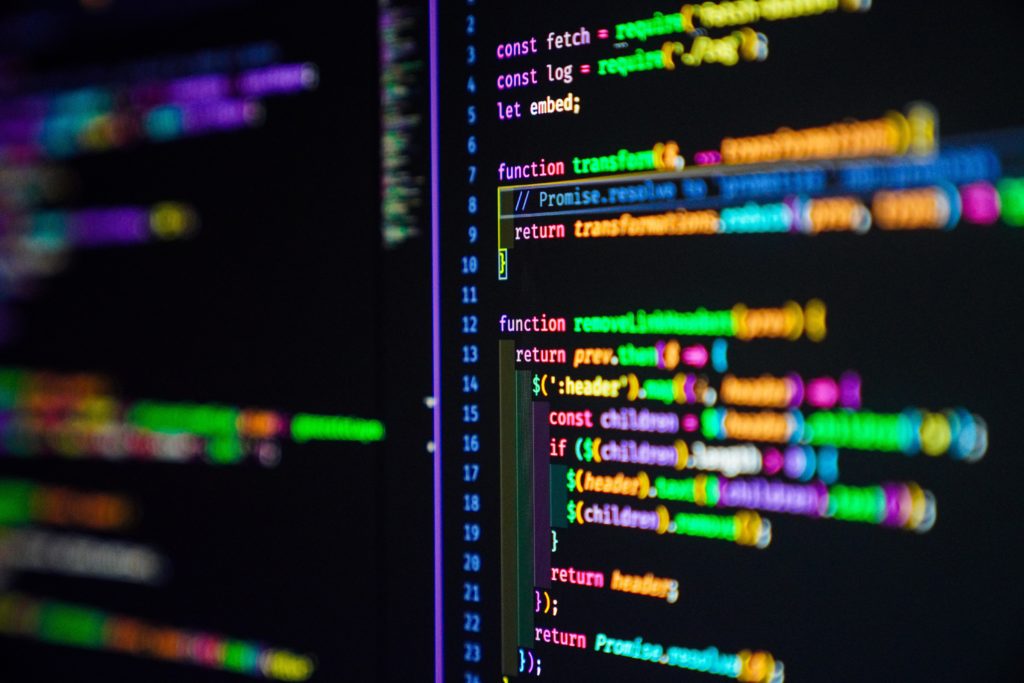
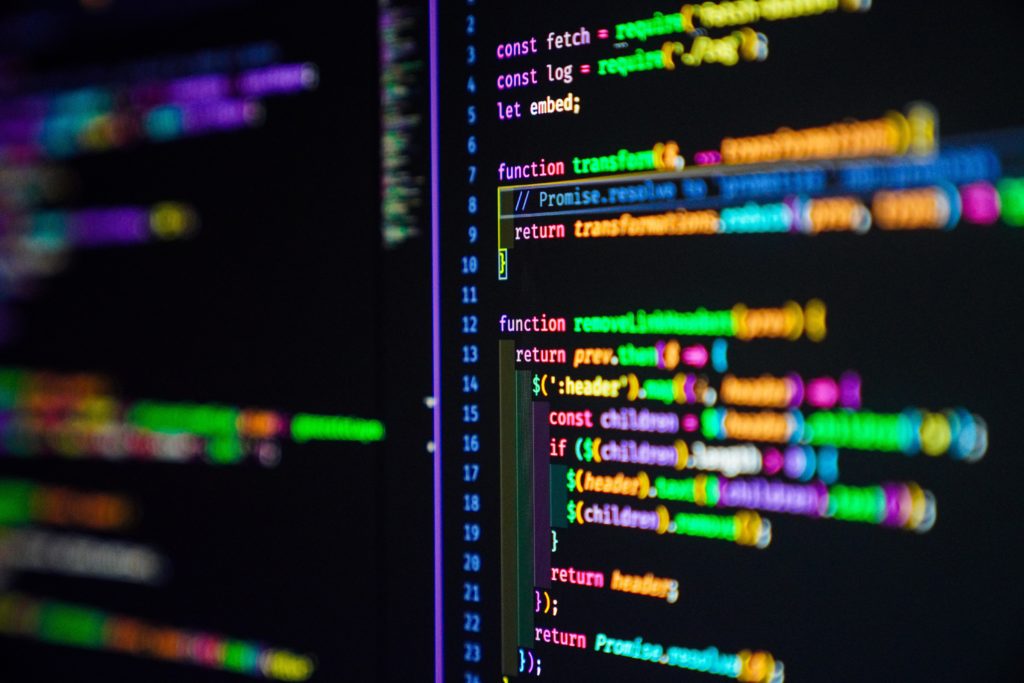
Functions in javascript
A function is a block of code that can be called by name. Functions are defined by using the keyword function, followed by the function’s name, followed by a set of parentheses; or by using arrow functions introduced with ES6. In both cases the parentheses contain the parameters that the function takes.
For example :
function sayHello() {
console.log("hello");
};
This means that sayHello is a function, that doesn’t have any arguments and will display “hello” in the console.
To execute this function, we need to call it :
sayHello();
First-class functions
First-class functions are functions that can be assigned to variables, passed as arguments to other functions, and returned from other functions. In JavaScript, all functions are first-class functions. This means that you can freely use functions as data values.
To assign a function to a variable in javascript:
const sayHello = function() {
console.log("Hello");
};
Or with an arrow function:
const sayHello = () => {
console.log("Hello");
};
First-class functions are an important part of Javascript. They allow you to treat functions like any other variable, which makes them more versatile and useful.
Higher order functions
Higher-order functions are functions that take other functions as arguments or return a function. These functions are very powerful and can be used to write concise and readable code. Higher-order functions can be used in many different situations, but they are particularly useful for iterating over data structures, building complex algorithms, and creating new abstractions. When used properly, higher-order functions can make your code more maintainable and easier to understand.
For example, the Array.map() method is a higher-order function that takes a callback function as an argument. The callback function is invoked once for each element in the array, and the return value is used to create a new array. In this way, map() can be used to transform an array of data into another form. Similarly, the Array.filter() method takes a callback function as an argument and returns a new array consisting of only those elements for which the callback returns true.
Exemple with array.map():
const myArray = [1, 2, 3, 4, 5];
newArray = myArray.map(item => item * 2);
console.log(newArray); // [2, 4, 5, 8, 10]
The map() function takes a function as argument that will multiply each item from the array by 2. The map() function returns a new array.
Returning functions from functions is a powerful way to create reusable code. For example, the following function takes two numbers as input and returns a function that multiplies those two numbers together:
const multiply = (x, y) => () => x * y;
This function can then be called like this:
multiply(3, 4); // returns a function
multiply(3, 4)(); // invokes the function and returns 12
This technique can be used to create many useful functions, such as a function that takes an array of numbers and returns a new array consisting of only the odd numbers:
const onlyOdd = numbers => numbers.filter(n => n % 2 === 1);
onlyOdd([1, 2, 3, 4, 5]); // returns only the odd numbers: 1, 3, 5
Similarly, a function that takes an array of numbers and multiplies them all together can be created like this:
const multiplyAll = numbers => numbers.reduce((acc, n) => acc * n);
multiplyAll([1, 2, 3]); // returns 6 (1 * 2 * 3)
As you can see, higher-order functions are a powerful tool that can be used to write concise and readable code. When used properly, they can make your code more maintainable and easier to understand. With that said, there are a few things to keep in mind when using higher-order functions.
First, make sure that the function you’re passing as an argument is pure (i.e., it doesn’t have any side effects).
Second, avoid creating nested higher-order functions, as this can make your code difficult to understand.
Finally, make sure that the return value of a higher-order function is used in some way, otherwise, it will be wasted.
Higher-order functions are an important part of functional programming, and they can be used to write concise and readable code.
Functional programming
Functional programming is a programming paradigm that emphasizes the evaluation of functions.
It is a declarative programming style that uses expressions and declarations instead of statements. Functional programming is a composition of many small functions.
The benefits of functional programming include code reuse, declarative coding, and easier debugging.
Functional programming can be used in javascript by using higher-order functions, first-class functions, and function closures. Higher-order functions take other functions as arguments or return them as results. First-class functions can be assigned to variables, members of objects, and passed as arguments to other functions. Function closures are inner functions that have access to the outer function’s variables.
Functional programming can help make code more concise and easier to read. It can also improve performance by making it easier to parallelize code.
When used properly, functional programming can make your code more maintainable and easier to understand. However, functional programming can also make your code more difficult to understand, so it is important to use it judiciously.
Conclusion
Higher-order functions are an important part of javascript and can be used to write concise and readable code. They are particularly useful for iterating over data structures, building complex algorithms, and creating new abstractions.
What can you do to help me?
Please don’t hesitate to:
- Like the article
- Follow me
- Leave a comment and express your opinion.
Happy coding!